300
|
How can I change the font for entire item
Dim f
With Excombobox1
.Columns.Add("Default")
.Items.AddItem("default font")
f = New stdole.StdFont()
With f
.Name = "Tahoma"
.Size = 12
End With
With .Items
.set_ItemFont(.AddItem("new font"),f)
End With
End With
|
299
|
How do I vertically align a cell
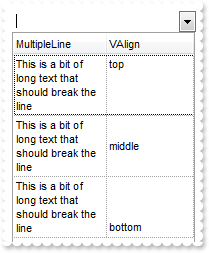
Dim h
With Excombobox1
.DrawGridLines = exontrol.EXCOMBOBOXLib.GridLinesEnum.exRowLines
.Columns.Add("MultipleLine").set_Def(exontrol.EXCOMBOBOXLib.DefColumnEnum.exCellSingleLine,False)
.Columns.Add("VAlign")
With .Items
h = .AddItem("This is a bit of long text that should break the line")
.set_CellCaption(h,1,"top")
.set_CellVAlignment(h,1,exontrol.EXCOMBOBOXLib.VAlignmentEnum.exTop)
h = .AddItem("This is a bit of long text that should break the line")
.set_CellCaption(h,1,"middle")
.set_CellVAlignment(h,1,exontrol.EXCOMBOBOXLib.VAlignmentEnum.exMiddle)
h = .AddItem("This is a bit of long text that should break the line")
.set_CellCaption(h,1,"bottom")
.set_CellVAlignment(h,1,exontrol.EXCOMBOBOXLib.VAlignmentEnum.exBottom)
End With
End With
|
298
|
How can I change the position of an item
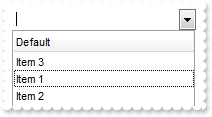
With Excombobox1
.Columns.Add("Default")
With .Items
.AddItem("Item 1")
.AddItem("Item 2")
.set_ItemPosition(.AddItem("Item 3"),0)
End With
End With
|
297
|
How do I find an item based on a path
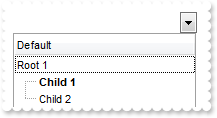
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.set_ItemData(.InsertItem(h,Nothing,"Child 2"),1234)
.set_ExpandItem(h,True)
.set_ItemBold(.get_FindPath("Root 1\Child 1"),True)
End With
End With
|
296
|
How do I find an item
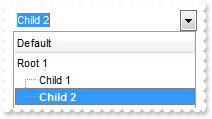
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.set_ItemBold(.get_FindItem("Child 2",0),True)
End With
End With
|
295
|
How can I insert a hyperlink or an anchor element
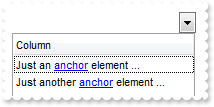
With Excombobox1
.Columns.Add("Column")
With .Items
.set_CellCaptionFormat(.AddItem("Just an <a1>anchor</a> element ..."),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML)
End With
With .Items
.set_CellCaptionFormat(.AddItem("Just another <a2>anchor</a> element ..."),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML)
End With
End With
|
294
|
How do I find the index of the item based on its handle
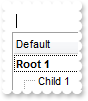
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.set_ItemBold(.get_ItemByIndex(.get_ItemToIndex(h)),True)
End With
End With
|
293
|
How do I find the handle of the item based on its index

Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.set_ItemBold(.get_ItemByIndex(1),True)
End With
End With
|
292
|
How can I find the cell being clicked in a radio group
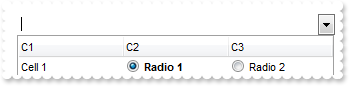
Dim h
With Excombobox1
.MarkSearchColumn = False
.SelBackColor = Color.FromArgb(255,255,128)
.SelForeColor = Color.FromArgb(0,0,0)
.Columns.Add("C1")
.Columns.Add("C2")
.Columns.Add("C3")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"Radio 1")
.set_CellHasRadioButton(h,1,True)
.set_CellRadioGroup(h,1,1234)
.set_CellCaption(h,2,"Radio 2")
.set_CellHasRadioButton(h,2,True)
.set_CellRadioGroup(h,2,1234)
.set_CellState(h,1,1)
.set_CellBold(Nothing,.get_CellChecked(1234),True)
End With
End With
|
291
|
Can I add a +/- ( expand / collapse ) buttons to each item, so I can load the child items later
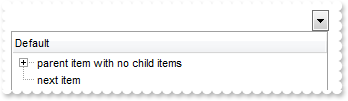
With Excombobox1
.LinesAtRoot = exontrol.EXCOMBOBOXLib.LinesAtRootEnum.exLinesAtRoot
.Columns.Add("Default")
With .Items
.set_ItemHasChildren(.AddItem("parent item with no child items"),True)
.AddItem("next item")
End With
End With
|
290
|
Can I let the user to resize at runtime the specified item
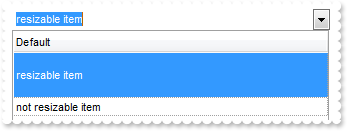
With Excombobox1
.ScrollBySingleLine = True
.DrawGridLines = exontrol.EXCOMBOBOXLib.GridLinesEnum.exRowLines
.Columns.Add("Default")
With .Items
.set_ItemAllowSizing(.AddItem("resizable item"),True)
.AddItem("not resizable item")
End With
End With
|
289
|
How can I change the size ( width, height ) of the picture
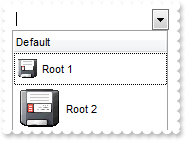
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.set_CellPicture(h,0,Excombobox1.ExecuteTemplate("loadpicture(`c:\exontrol\images\zipdisk.gif`)"))
.set_CellPictureWidth(h,0,24)
.set_CellPictureHeight(h,0,24)
.set_ItemHeight(h,32)
h = .AddItem("Root 2")
.set_CellPicture(h,0,Excombobox1.ExecuteTemplate("loadpicture(`c:\exontrol\images\zipdisk.gif`)"))
.set_ItemHeight(h,48)
End With
End With
|
288
|
How do I unselect an item
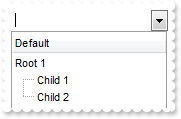
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.set_SelectItem(h,False)
End With
End With
|
287
|
How do I find the selected item
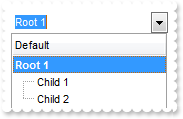
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.set_SelectItem(h,True)
.set_ItemBold(.get_SelectedItem(0),True)
End With
End With
|
286
|
How do I select an item
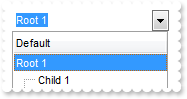
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.set_SelectItem(h,True)
End With
End With
|
285
|
Can I display a button with some picture or icon inside
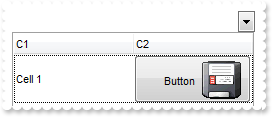
Dim h
With Excombobox1
.set_HTMLPicture("p1","c:\exontrol\images\zipdisk.gif")
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1," Button <img>p1</img> ")
.set_CellCaptionFormat(h,1,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML)
.set_CellHAlignment(h,1,exontrol.EXCOMBOBOXLib.AlignmentEnum.RightAlignment)
.set_CellHasButton(h,1,True)
.set_ItemHeight(h,48)
End With
End With
|
284
|
Can I display a button with some picture or icon inside
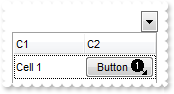
Dim h
With Excombobox1
.Images("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq" & _
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" & _
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" & _
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=")
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1," Button <img>1</img> ")
.set_CellCaptionFormat(h,1,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML)
.set_CellHAlignment(h,1,exontrol.EXCOMBOBOXLib.AlignmentEnum.RightAlignment)
.set_CellHasButton(h,1,True)
End With
End With
|
283
|
Can I display a button with some icon inside
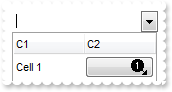
Dim h
With Excombobox1
.Images("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq" & _
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" & _
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" & _
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=")
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1," <img>1</img> ")
.set_CellCaptionFormat(h,1,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML)
.set_CellHAlignment(h,1,exontrol.EXCOMBOBOXLib.AlignmentEnum.RightAlignment)
.set_CellHasButton(h,1,True)
End With
End With
|
282
|
How can I assign multiple icon/picture to a cell
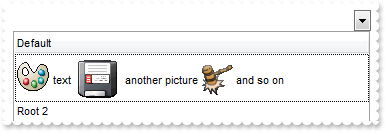
Dim h
With Excombobox1
.set_HTMLPicture("p1","c:\exontrol\images\zipdisk.gif")
.set_HTMLPicture("p2","c:\exontrol\images\auction.gif")
.Columns.Add("Default")
With .Items
h = .AddItem("text <img>p1</img> another picture <img>p2</img> and so on")
.set_CellCaptionFormat(h,0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML)
.set_CellPicture(h,0,Excombobox1.ExecuteTemplate("loadpicture(`c:\exontrol\images\colorize.gif`)"))
.set_ItemHeight(h,48)
.AddItem("Root 2")
End With
End With
|
281
|
How can I assign an icon/picture to a cell
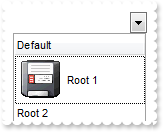
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.set_CellPicture(h,0,Excombobox1.ExecuteTemplate("loadpicture(`c:\exontrol\images\zipdisk.gif`)"))
.set_ItemHeight(h,48)
.AddItem("Root 2")
End With
End With
|
280
|
How can I assign multiple icons/pictures to a cell
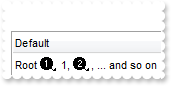
Dim h
With Excombobox1
.Images("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq" & _
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" & _
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" & _
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=")
.Columns.Add("Default")
With .Items
h = .AddItem("Root <img>1</img> 1, <img>2</img>, ... and so on ")
.set_CellCaptionFormat(h,0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML)
End With
End With
|
279
|
How can I assign multiple icons/pictures to a cell
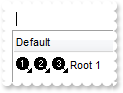
Dim h
With Excombobox1
.Images("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq" & _
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" & _
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" & _
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=")
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.set_CellImages(h,0,"1,2,3")
End With
End With
|
278
|
How can I assign an icon/picture to a cell
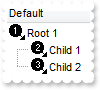
Dim h
With Excombobox1
.Images("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq" & _
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" & _
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" & _
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=")
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.set_CellImage(h,0,1)
.set_CellImage(.InsertItem(h,Nothing,"Child 1"),0,2)
.set_CellImage(.InsertItem(h,Nothing,"Child 2"),0,3)
.set_ExpandItem(h,True)
End With
End With
|
277
|
How can I get the handle of an item based on the handle of the cell
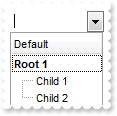
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.set_ItemBold(.get_CellItem(.get_ItemCell(h,0)),True)
End With
End With
|
276
|
How can I display a button inside the item or cell
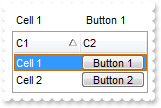
Dim h
With Excombobox1
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1," Button 1 ")
.set_CellHAlignment(h,1,exontrol.EXCOMBOBOXLib.AlignmentEnum.RightAlignment)
.set_CellHasButton(h,1,True)
h = .AddItem("Cell 2")
.set_CellCaption(h,1," Button 2 ")
.set_CellHAlignment(h,1,exontrol.EXCOMBOBOXLib.AlignmentEnum.CenterAlignment)
.set_CellHasButton(h,1,True)
End With
End With
|
275
|
How can I change the state of a radio button
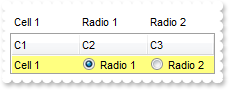
Dim h
With Excombobox1
.MarkSearchColumn = False
.SelBackColor = Color.FromArgb(255,255,128)
.SelForeColor = Color.FromArgb(0,0,0)
.Columns.Add("C1")
.Columns.Add("C2")
.Columns.Add("C3")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"Radio 1")
.set_CellHasRadioButton(h,1,True)
.set_CellRadioGroup(h,1,1234)
.set_CellCaption(h,2,"Radio 2")
.set_CellHasRadioButton(h,2,True)
.set_CellRadioGroup(h,2,1234)
.set_CellState(h,1,1)
End With
End With
|
274
|
How can I assign a radio button to a cell
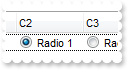
Dim h
With Excombobox1
.MarkSearchColumn = False
.SelBackColor = Color.FromArgb(255,255,128)
.SelForeColor = Color.FromArgb(0,0,0)
.Columns.Add("C1")
.Columns.Add("C2")
.Columns.Add("C3")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"Radio 1")
.set_CellHasRadioButton(h,1,True)
.set_CellRadioGroup(h,1,1234)
.set_CellCaption(h,2,"Radio 2")
.set_CellHasRadioButton(h,2,True)
.set_CellRadioGroup(h,2,1234)
.set_CellState(h,1,1)
End With
End With
|
273
|
How can I change the state of a checkbox
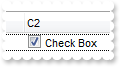
Dim h
With Excombobox1
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"Check Box")
.set_CellHasCheckBox(h,1,True)
.set_CellState(h,1,1)
End With
End With
|
272
|
How can I assign a checkbox to a cell
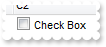
Dim h
With Excombobox1
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"Check Box")
.set_CellHasCheckBox(h,1,True)
End With
End With
|
271
|
How can I display an item or a cell on multiple lines
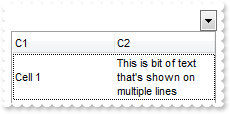
Dim h
With Excombobox1
.ScrollBySingleLine = True
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"This is bit of text that's shown on multiple lines")
.set_CellSingleLine(h,1,exontrol.EXCOMBOBOXLib.CellSingleLineEnum.exCaptionWordWrap)
End With
End With
|
270
|
How can I assign a tooltip to a cell
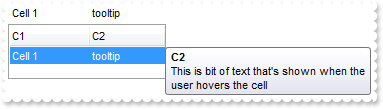
Dim h
With Excombobox1
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"tooltip")
.set_CellToolTip(h,1,"This is bit of text that's shown when the user hovers the cell")
End With
End With
|
269
|
How can I associate an extra data to a cell
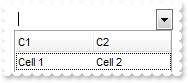
Dim h
With Excombobox1
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"Cell 2")
.set_CellData(h,1,"your extra data")
End With
End With
|
268
|
How do I enable or disable a cell
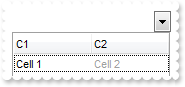
Dim h
With Excombobox1
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"Cell 2")
.set_CellEnabled(h,1,False)
End With
End With
|
267
|
How do I change the cell's foreground color
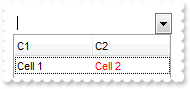
Dim h
With Excombobox1
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"Cell 2")
.set_CellForeColor(h,1,Color.FromArgb(255,0,0))
End With
End With
|
266
|
How do I change the visual effect for the cell, using your EBN files
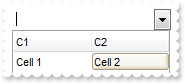
Dim h
With Excombobox1
.VisualAppearance.Add(1,"c:\exontrol\images\normal.ebn")
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"Cell 2")
.set_CellBackColor32(h,1,&H1000000)
End With
End With
|
265
|
How do I change the cell's background color
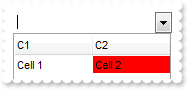
Dim h
With Excombobox1
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"Cell 2")
.set_CellBackColor(h,1,Color.FromArgb(255,0,0))
End With
End With
|
264
|
How do I change the caption or value for a particular cell
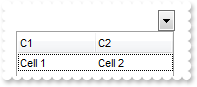
With Excombobox1
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
.set_CellCaption(.AddItem("Cell 1"),1,"Cell 2")
End With
End With
|
263
|
How do I get the handle of the cell
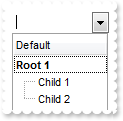
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.set_CellBold(Nothing,.get_ItemCell(h,0),True)
End With
End With
|
262
|
How do I retrieve the focused item
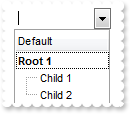
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.set_ItemBold(.FocusItem,True)
End With
End With
|
261
|
How do I get the number or count of child items
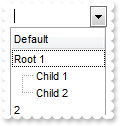
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.AddItem(.get_ChildCount(h))
End With
End With
|
260
|
How do I enumerate the visible items
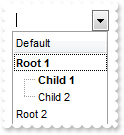
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
h = .AddItem("Root 2")
.set_ItemBold(.FirstVisibleItem,True)
.set_ItemBold(.get_NextVisibleItem(.FirstVisibleItem),True)
End With
End With
|
259
|
How do I enumerate the siblings items
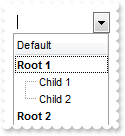
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
h = .AddItem("Root 2")
.set_ItemBold(.get_NextSiblingItem(.FirstVisibleItem),True)
.set_ItemBold(.get_PrevSiblingItem(.get_NextSiblingItem(.FirstVisibleItem)),True)
End With
End With
|
258
|
How do I get the parent item
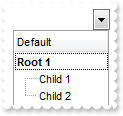
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.set_ItemBold(.get_ItemParent(.get_ItemChild(h)),True)
End With
End With
|
257
|
How do I get the first child item
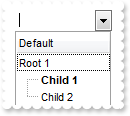
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.set_ItemBold(.get_ItemChild(h),True)
End With
End With
|
256
|
How do I enumerate the root items
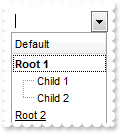
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
h = .AddItem("Root 2")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ItemBold(.get_RootItem(0),True)
.set_ItemUnderline(.get_RootItem(1),True)
End With
End With
|
255
|
I have a hierarchy, how can I count the number of root items
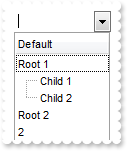
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root 1")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
h = .AddItem("Root 2")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.AddItem(.RootCount)
End With
End With
|
254
|
How can I make an item unselectable, or not selectable
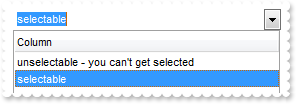
Dim h
With Excombobox1
.Columns.Add("Column")
With .Items
h = .AddItem("unselectable - you can't get selected")
.set_SelectableItem(h,False)
.AddItem("selectable")
End With
End With
|
253
|
How can I hide or show an item
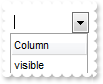
Dim h
With Excombobox1
.Columns.Add("Column")
With .Items
h = .AddItem("hidden")
.set_ItemHeight(h,0)
.set_SelectableItem(h,False)
.AddItem("visible")
End With
End With
|
252
|
How can I change the height for all items
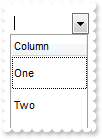
With Excombobox1
.DefaultItemHeight = 32
.Columns.Add("Column")
.Items.AddItem("One")
.Items.AddItem("Two")
End With
|
251
|
How do I change the height of an item
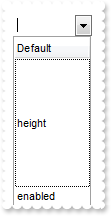
With Excombobox1
.ScrollBySingleLine = True
.Columns.Add("Default")
With .Items
.set_ItemHeight(.AddItem("height"),128)
End With
.Items.AddItem("enabled")
End With
|
250
|
How do I disable or enable an item
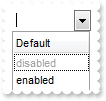
With Excombobox1
.Columns.Add("Default")
With .Items
.set_EnableItem(.AddItem("disabled"),False)
End With
.Items.AddItem("enabled")
End With
|
249
|
How do I display as strikeout a cell
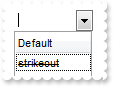
With Excombobox1
.Columns.Add("Default")
With .Items
.set_CellStrikeOut(.AddItem("strikeout"),0,True)
End With
End With
|
248
|
How do I display as strikeout a cell or an item
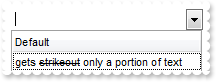
With Excombobox1
.Columns.Add("Default")
With .Items
.set_CellCaptionFormat(.AddItem("gets <s>strikeout</s> only a portion of text"),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML)
End With
End With
|
247
|
How do I display as strikeout an item
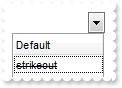
With Excombobox1
.Columns.Add("Default")
With .Items
.set_ItemStrikeOut(.AddItem("strikeout"),True)
End With
End With
|
246
|
How do I underline a cell
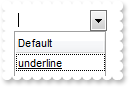
With Excombobox1
.Columns.Add("Default")
With .Items
.set_CellUnderline(.AddItem("underline"),0,True)
End With
End With
|
245
|
How do I underline a cell or an item
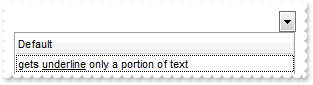
With Excombobox1
.Columns.Add("Default")
With .Items
.set_CellCaptionFormat(.AddItem("gets <u>underline</u> only a portion of text"),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML)
End With
End With
|
244
|
How do I underline an item
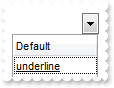
With Excombobox1
.Columns.Add("Default")
With .Items
.set_ItemUnderline(.AddItem("underline"),True)
End With
End With
|
243
|
How do I display as italic a cell
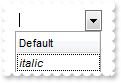
With Excombobox1
.Columns.Add("Default")
With .Items
.set_CellItalic(.AddItem("italic"),0,True)
End With
End With
|
242
|
How do I display as italic a cell or an item
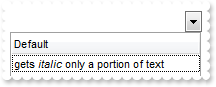
With Excombobox1
.Columns.Add("Default")
With .Items
.set_CellCaptionFormat(.AddItem("gets <i>italic</i> only a portion of text"),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML)
End With
End With
|
241
|
How do I display as italic an item

With Excombobox1
.Columns.Add("Default")
With .Items
.set_ItemItalic(.AddItem("italic"),True)
End With
End With
|
240
|
How do I bold a cell

With Excombobox1
.Columns.Add("Default")
With .Items
.set_CellBold(.AddItem("bold"),0,True)
End With
End With
|
239
|
How do I bold a cell or an item
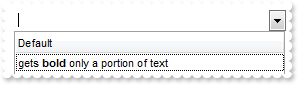
With Excombobox1
.Columns.Add("Default")
With .Items
.set_CellCaptionFormat(.AddItem("gets <b>bold</b> only a portion of text"),0,exontrol.EXCOMBOBOXLib.CaptionFormatEnum.exHTML)
End With
End With
|
238
|
How do I bold an item
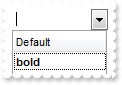
With Excombobox1
.Columns.Add("Default")
With .Items
.set_ItemBold(.AddItem("bold"),True)
End With
End With
|
237
|
How do I change the foreground color for the item
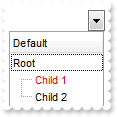
Dim h,hC
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root")
hC = .InsertItem(h,Nothing,"Child 1")
.set_ItemForeColor(hC,Color.FromArgb(255,0,0))
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
End With
End With
|
236
|
How do I change the visual appearance for the item, using your EBN technology
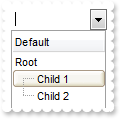
Dim h,hC
With Excombobox1
.VisualAppearance.Add(1,"c:\exontrol\images\normal.ebn")
.Columns.Add("Default")
With .Items
h = .AddItem("Root")
hC = .InsertItem(h,Nothing,"Child 1")
.set_ItemBackColor32(hC,&H1000000)
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
End With
End With
|
235
|
How do I change the background color for the item
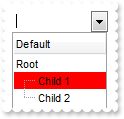
Dim h,hC
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root")
hC = .InsertItem(h,Nothing,"Child 1")
.set_ItemBackColor(hC,Color.FromArgb(255,0,0))
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
End With
End With
|
234
|
How do I expand or collapse an item
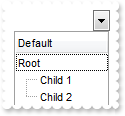
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
End With
End With
|
233
|
How do I associate an extra data to an item
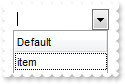
With Excombobox1
.Columns.Add("Default")
With .Items
.set_ItemData(.AddItem("item"),"your extra data")
End With
End With
|
232
|
How do I get the number or count of items
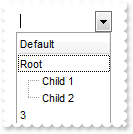
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
End With
With .Items
.AddItem(.ItemCount)
End With
End With
|
231
|
How can I change at runtime the parent of the item
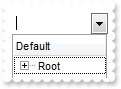
Dim hC,hP
With Excombobox1
.LinesAtRoot = exontrol.EXCOMBOBOXLib.LinesAtRootEnum.exLinesAtRoot
.Columns.Add("Default")
With .Items
hP = .AddItem("Root")
hC = .AddItem("Child")
.SetParent(hC,hP)
End With
End With
|
230
|
How can I sort the items
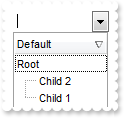
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
End With
.Columns.Item("Default").SortOrder = exontrol.EXCOMBOBOXLib.SortOrderEnum.SortDescending
End With
|
229
|
How do I sort the child items
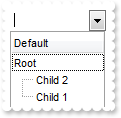
Dim h
With Excombobox1
.Columns.Add("Default")
With .Items
h = .AddItem("Root")
.InsertItem(h,Nothing,"Child 1")
.InsertItem(h,Nothing,"Child 2")
.set_ExpandItem(h,True)
.SortChildren(h,0,False)
End With
End With
|
228
|
How can I remove or delete all items
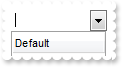
With Excombobox1
.Columns.Add("Default")
.Items.AddItem("removed item")
.Items.RemoveAllItems()
End With
|
227
|
How can I remove or delete an item
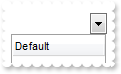
Dim h
With Excombobox1
.Columns.Add("Default")
h = .Items.AddItem("removed item")
.Items.RemoveItem(h)
End With
|
226
|
How can I add or insert child items
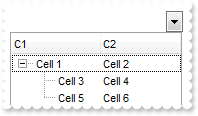
Dim h
With Excombobox1
.LinesAtRoot = exontrol.EXCOMBOBOXLib.LinesAtRootEnum.exLinesAtRoot
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
h = .AddItem("Cell 1")
.set_CellCaption(h,1,"Cell 2")
.set_CellCaption(.InsertItem(h,Nothing,"Cell 3"),1,"Cell 4")
.set_CellCaption(.InsertItem(h,Nothing,"Cell 5"),1,"Cell 6")
.set_ExpandItem(h,True)
End With
End With
|
225
|
How can I add or insert a child item
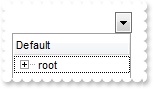
With Excombobox1
.LinesAtRoot = exontrol.EXCOMBOBOXLib.LinesAtRootEnum.exLinesAtRoot
.Columns.Add("Default")
With .Items
.InsertItem(.AddItem("root"),Nothing,"child")
End With
End With
|
224
|
How can I add or insert an item
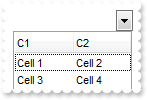
Dim h
With Excombobox1
.Columns.Add("C1")
.Columns.Add("C2")
With .Items
.set_CellCaption(.AddItem("Cell 1"),1,"Cell 2")
h = .AddItem("Cell 3")
.set_CellCaption(h,1,"Cell 4")
End With
End With
|
223
|
How can I add or insert an item
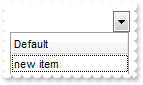
With Excombobox1
.Columns.Add("Default")
.Items.AddItem("new item")
End With
|
222
|
How can I get the columns as they are shown in the control's sortbar
Dim var_Object
With Excombobox1
var_Object = .Columns.get_ItemBySortPosition(0)
End With
|
221
|
How can I access the properties of a column
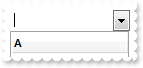
With Excombobox1
.Columns.Add("A")
.Columns.Item("A").HeaderBold = True
End With
|
220
|
How can I remove all the columns
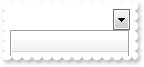
With Excombobox1
.Columns.Clear()
End With
|
219
|
How can I remove a column
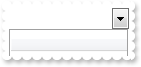
With Excombobox1
.Columns.Remove("A")
End With
|
218
|
How can I get the number or the count of columns
Dim var_Count
With Excombobox1
var_Count = .Columns.Count
End With
|
217
|
How can I change the font for all cells in the entire column
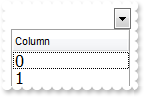
Dim f
With Excombobox1
f = New stdole.StdFont()
With f
.Name = "Tahoma"
.Size = 12
End With
With .ConditionalFormats.Add("1")
.Font = f
.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns
End With
.Columns.Add("Column")
.Items.AddItem(0)
.Items.AddItem(1)
End With
|
216
|
How can I change the background color for all cells in the column
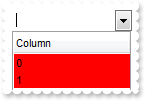
Dim var_ConditionalFormat
With Excombobox1
var_ConditionalFormat = .ConditionalFormats.Add("1")
With var_ConditionalFormat
.BackColor = Color.FromArgb(255,0,0)
.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns
End With
.Columns.Add("Column")
.Items.AddItem(0)
.Items.AddItem(1)
End With
|
215
|
How can I change the foreground color for all cells in the column
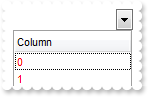
Dim var_ConditionalFormat
With Excombobox1
var_ConditionalFormat = .ConditionalFormats.Add("1")
With var_ConditionalFormat
.ForeColor = Color.FromArgb(255,0,0)
.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns
End With
.Columns.Add("Column")
.Items.AddItem(0)
.Items.AddItem(1)
End With
|
214
|
How can I show as strikeout all cells in the column
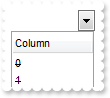
Dim var_ConditionalFormat
With Excombobox1
var_ConditionalFormat = .ConditionalFormats.Add("1")
With var_ConditionalFormat
.StrikeOut = True
.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns
End With
.Columns.Add("Column")
.Items.AddItem(0)
.Items.AddItem(1)
End With
|
213
|
How can I underline all cells in the column
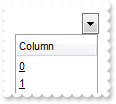
Dim var_ConditionalFormat
With Excombobox1
var_ConditionalFormat = .ConditionalFormats.Add("1")
With var_ConditionalFormat
.Underline = True
.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns
End With
.Columns.Add("Column")
.Items.AddItem(0)
.Items.AddItem(1)
End With
|
212
|
How can I show in italic all data in the column
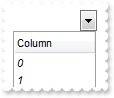
Dim var_ConditionalFormat
With Excombobox1
var_ConditionalFormat = .ConditionalFormats.Add("1")
With var_ConditionalFormat
.Italic = True
.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns
End With
.Columns.Add("Column")
.Items.AddItem(0)
.Items.AddItem(1)
End With
|
211
|
How can I bold the entire column
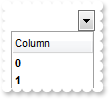
Dim var_ConditionalFormat
With Excombobox1
var_ConditionalFormat = .ConditionalFormats.Add("1")
With var_ConditionalFormat
.Bold = True
.ApplyTo = exontrol.EXCOMBOBOXLib.FormatApplyToEnum.exFormatToColumns
End With
.Columns.Add("Column")
.Items.AddItem(0)
.Items.AddItem(1)
End With
|
210
|
How can I display a computed column and highlight some values that are negative or less than a value
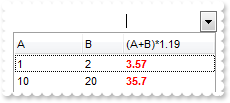
Dim var_ConditionalFormat
With Excombobox1
.Columns.Add("A")
.Columns.Add("B")
.Columns.Add("(A+B)*1.19").ComputedField = "(%0 + %1) * 1.19"
With .Items
.set_CellCaption(.AddItem(1),1,2)
End With
With .Items
.set_CellCaption(.AddItem(10),1,20)
End With
var_ConditionalFormat = .ConditionalFormats.Add("%2 > 10")
With var_ConditionalFormat
.Bold = True
.ForeColor = Color.FromArgb(255,0,0)
.ApplyTo = &H2
End With
End With
|
209
|
Can I display a computed column so it displays the VAT, or SUM
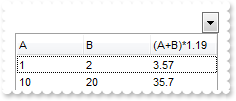
With Excombobox1
.Columns.Add("A")
.Columns.Add("B")
.Columns.Add("(A+B)*1.19").ComputedField = "(%0 + %1) * 1.19"
With .Items
.set_CellCaption(.AddItem(1),1,2)
End With
With .Items
.set_CellCaption(.AddItem(10),1,20)
End With
End With
|
208
|
How can I show a column that adds values in the cells
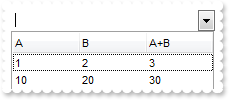
With Excombobox1
.Columns.Add("A")
.Columns.Add("B")
.Columns.Add("A+B").ComputedField = "%0 + %1"
With .Items
.set_CellCaption(.AddItem(1),1,2)
End With
With .Items
.set_CellCaption(.AddItem(10),1,20)
End With
End With
|
207
|
Is there any function to filter the control's data as I type, so the items being displayed include the typed characters
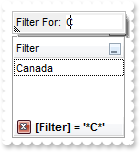
Dim var_Column
With Excombobox1
var_Column = .Columns.Add("Filter")
With var_Column
.FilterOnType = True
.DisplayFilterButton = True
.AutoSearch = exontrol.EXCOMBOBOXLib.AutoSearchEnum.exContains
End With
.Items.AddItem("Canada")
.Items.AddItem("USA")
End With
|
206
|
Is there any function to filter the control's data as I type, something like filter on type
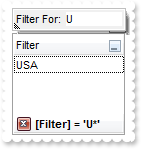
Dim var_Column
With Excombobox1
var_Column = .Columns.Add("Filter")
With var_Column
.FilterOnType = True
.DisplayFilterButton = True
End With
.Items.AddItem("Canada")
.Items.AddItem("USA")
End With
|
205
|
How can I programmatically filter a column
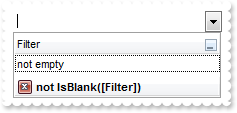
With Excombobox1
With .Columns.Add("Filter")
.DisplayFilterButton = True
.FilterType = exontrol.EXCOMBOBOXLib.FilterTypeEnum.exNonBlanks
End With
.Items.AddItem()
.Items.AddItem("not empty")
.ApplyFilter()
End With
|
204
|
How can I show or display the control's filter
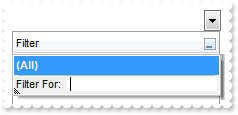
With Excombobox1
.Columns.Add("Filter").DisplayFilterButton = True
End With
|
203
|
How can I customize the items being displayed in the drop down filter window
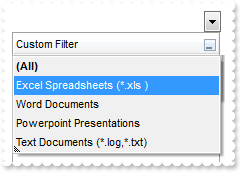
With Excombobox1
With .Columns.Add("Custom Filter")
.DisplayFilterButton = True
.DisplayFilterPattern = False
.CustomFilter = "Excel Spreadsheets (*.xls )||*.xls|||Word Documents||*.doc|||Powerpoint Presentations||*.pps|||Text Documents (*.log,*.txt)||*." & _
"txt|*.log"
.FilterType = exontrol.EXCOMBOBOXLib.FilterTypeEnum.exPattern
.Filter = "*.xls"
End With
.Items.AddItem("excel.xls")
.Items.AddItem("word.doc")
.Items.AddItem("pp.pps")
.Items.AddItem("text.txt")
.ApplyFilter()
End With
|
202
|
How can I change the order or the position of the columns in the sort bar
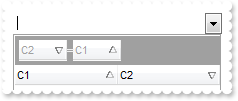
With Excombobox1
.SortBarVisible = True
.SortBarColumnWidth = 48
.Columns.Add("C1").SortOrder = exontrol.EXCOMBOBOXLib.SortOrderEnum.SortAscending
.Columns.Add("C2").SortOrder = exontrol.EXCOMBOBOXLib.SortOrderEnum.SortDescending
.Columns.Item("C2").SortPosition = 0
End With
|
201
|
How do I arrange my columns on multiple levels
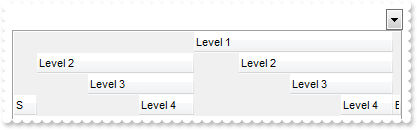
With Excombobox1
.Columns.Add("S").Width = 32
.Columns.Add("Level 2").LevelKey = 1
.Columns.Add("Level 3").LevelKey = 1
.Columns.Add("Level 4").LevelKey = 1
.Columns.Add("Level 1").LevelKey = "2"
.Columns.Add("Level 2").LevelKey = "2"
.Columns.Add("Level 3").LevelKey = "2"
.Columns.Add("Level 4").LevelKey = "2"
.Columns.Add("E").Width = 32
End With
|